c++ programming language
C++ is a cross-platform language that can be used to create high-performance applications. C++ was developed by Bjarne Stroustrup, as an extension to the C language. C++ gives programmers a high level of control over system resources and memory.
About c++ programming language |
What is C++?
![]() |
What is c++ in programming |
C++ is a cross-platform language that can be used to create high-performance applications.
C++ was developed by Bjarne Stroustrup, as an extension to the C language.
C++ gives programmers a high level of control over system resources and memory.
The language was updated 3 major times in 2011, 2014, and 2017 to C++11, C++14, and C++17.
Why Use C++
![]() |
Why use c++ for games |
C++ is one of the world's most popular programming languages.
C++ can be found in today's operating systems, Graphical User Interfaces, and embedded systems.
C++ is an object-oriented programming language which gives a clear structure to programs and allows code to be reused, lowering development costs.
C++ is portable and can be used to develop applications that can be adapted to multiple platforms.
C++ is fun and easy to learn!
C++ Install IDE
An IDE (Integrated Development Environment) is used to edit AND compile the code.
Popular IDE's include Code::Blocks, Eclipse, and Visual Studio. These are all free, and they can be used to both edit and debug C++ code.
Note: Web-based IDE's can work as well, but functionality is limited.
We will use Code::Blocks in our tutorial, which we believe is a good place to start.
You can find the latest version of Codeblocks at http://www.codeblocks.org/downloads/26. Download the
mingw-setup.exe
file, which will install the text editor with a compiler.myfirstprogram.cpp
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!";
return 0;
}
RESULT :
Hello world
C++ Comments
![]() |
C++ comment section |
Comments can be used to explain C++ code, and to make it more readable. It can also be used to prevent execution when testing alternative code. Comments can be singled-lined or multi-lined.
Single-line comments start with two forward slashes (
//
).
Any text between
//
and the end of the line is ignored by the compiler (will not be executed).
This example uses a single-line comment before a line of code:
Example
// This is a commentcout << "Hello World!";
C++ Variables
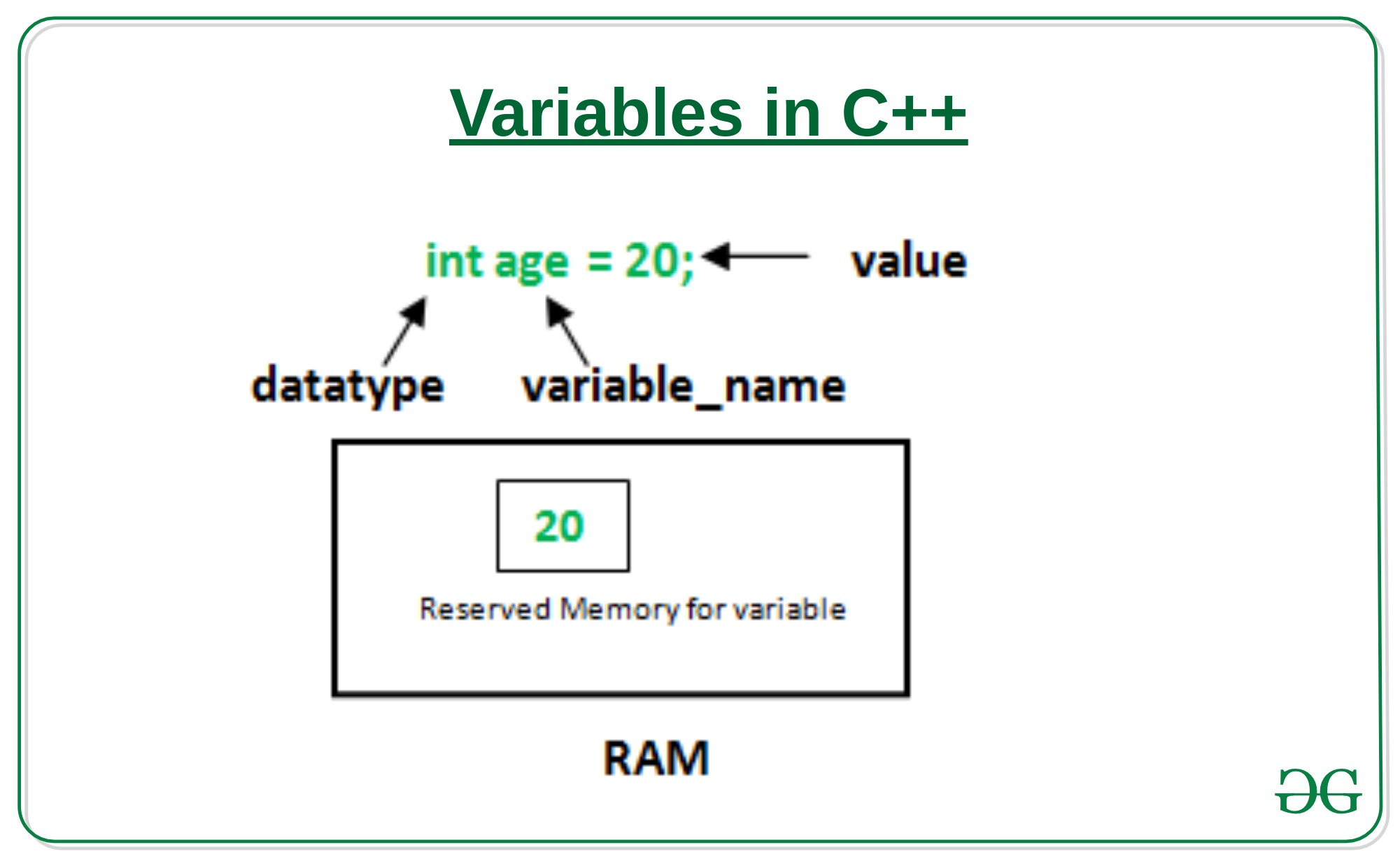
c++ variables
Variables are containers for storing data values.
In C++, there are different types of variables (defined with different keywords), for example:
int
- stores integers (whole numbers), without decimals, such as 123 or -123
double
- stores floating point numbers, with decimals, such as 19.99 or -19.99
char
- stores single characters, such as 'a' or 'B'. Char values are surrounded by single quotes
Declaring (Creating) Variables
To create a variable, you must specify the type and assign it a value:
syntax
type variable = value;
Where type is one of C++ types (such as int
), and variable is the name of the variable (such as x or myName). The equal sign is used to assign values to the variable.
Display Variables
The cout
object is used together with the <<
operator to display variables.
To combine both text and a variable, separate them with the <<
operator:
int myAge = 35;
cout << "I am " << myAge << " years old.";
Add Variables Together
To add a variable to another variable, you can use the +
operator:
int x = 5;
int y = 6;
int sum = x + y;
cout << sum;
Declare Many Variables
To declare more than one variable of the same type, use a comma-separated list:
int x = 5, y = 6, z = 50;
cout << x + y + z;
C++ Identifiers
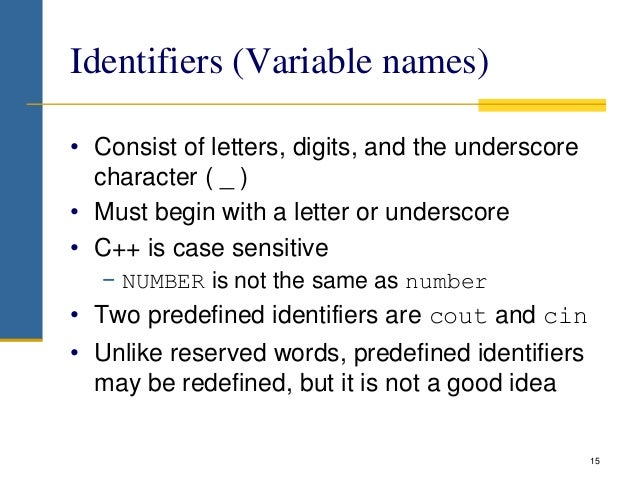
c++ identifiers
All C++ variables must be identified with unique names.
These unique names are called identifiers.
Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume).
// Goodint minutesPerHour = 60;
// OK, but not so easy to understand what m actually isint m = 60;
The general rules for constructing names for variables (unique identifiers) are:
- Names can contain letters, digits and underscores
- Names must begin with a letter or an underscore (_)
- Names are case sensitive (
myVar
and myvar
are different variables)
- Names cannot contain whitespaces or special characters like !, #, %, etc.
- Reserved words (like C++ keywords, such as
int
) cannot be used as names.
Constants
When you do not want others (or yourself) to override existing variable values, use the const
keyword (this will declare the variable as "constant", which means unchangeable and read-only):
const int myNum = 15; // myNum will always be 15myNum = 10; // error: assignment of read-only variable 'myNum'
C++ User Input
You have already learned that cout
is used to output (print) values. Now we will use cin
to get user input.
cin
is a predefined variable that reads data from the keyboard with the extraction operator (>>
).
In the following example, the user can input a number, which is stored in the variable x
. Then we print the value of x
:
int x;
cout << "Type a number: "; // Type a number and press entercin >> x; // Get user input from the keyboardcout << "Your number is: " << x; // Display the input value
cout
is pronounced "see-out". Used for output, and uses the insertion operator (<<
)
cin
is pronounced "see-in". Used for input, and uses the extraction operator (>>
)
C++ Data Types
As explained in the Variables chapter, a variable in C++ must be a specified data type:
Basic Data Types

basic datatypes in c++
The data type specifies the size and type of information the variable will store:
Data Type Size Description
int
4 bytes Stores whole numbers, without decimals
float
4 bytes Stores fractional numbers, containing one or more decimals. Sufficient for storing 7 decimal digits
double
8 bytes Stores fractional numbers, containing one or more decimals. Sufficient for storing 15 decimal digits
boolean
1 byte Stores true or false values
char
1 byte Stores a single character/letter/number, or ASCII values
Numeric Types
Use int
when you need to store a whole number without decimals, like 35 or 1000, and float
or double
when you need a floating point number (with decimals), like 9.99 or 3.14515.
int myNum = 1000;
cout << myNum;
Scientific Numbers
A floating point number can also be a scientific number with an "e" to indicate the power of 10:
float f1 = 35e3;
double d1 = 12E4;
cout << f1;
cout << d1;
Boolean Types
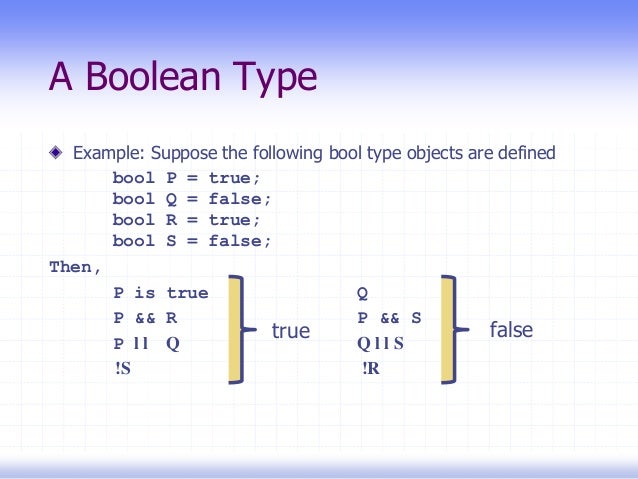
boolean type c++
bool isCodingFun = true;
bool isFishTasty = false;
cout << isCodingFun; // Outputs 1 (true)cout << isFishTasty; // Outputs 0 (false)
Character Types
The char
data type is used to store a single character. The character must be surrounded by single quotes, like 'A' or 'c':
char myGrade = 'B';
cout << myGrade;
Alternatively, you can use ASCII values to display certain characters:
char a = 65, b = 66, c = 67;
cout << a;
cout << b;
cout << c;